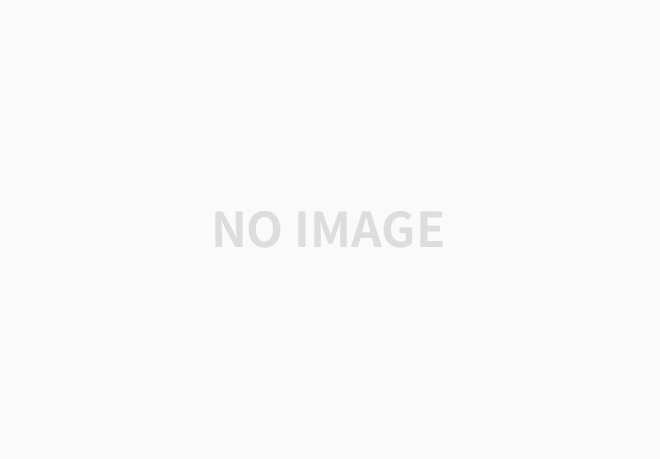
https://typescript-kr.github.io/pages/Classes.html
TypeScript 한글 문서
TypeScript 한글 번역 문서입니다
typescript-kr.github.io
타입스크립트 알짜배기 정리
- 클래스
- 간단한 클래스를 만들어보자.
class Waist {
xscope : number;
yscope : number;
readonly max : number = 100; // 스파르타 우! 우!
constructor(x : number, y : number){
this.xscope = x;
this.yscope = y;
}
turnAround() {
for(let angle = 0; angle <= 360; angle++){
const x = (Math.cos(this.degrees_to_radians(angle)) * this.max ) + this.xscope;
const y = (Math.sin(this.degrees_to_radians(angle)) * this.max ) + this.yscope;
console.log(parseInt(x.toString()), parseInt(y.toString()));
}
}
degrees_to_radians(degrees)
{
return degrees * (Math.PI/180);
}
}
let myWaist = new Waist(0, 0);
myWaist.turnAround(); // 아 내 허리는 지금 360가 안 돌아간다. 코드라도 돌아가라. 석고보드 800개 옮기기 ㅅㅂ
- 상속
- 상속이 정말 객체지향인 것인가?
- 의존성 주입 만세!!!
- 어째든 상속해보자.
- 상속이 정말 객체지향인 것인가?
class DontInheritMe {
Say(){
console.log('너가 상속하면 안되지!');
}
}
class IWillIngeriteYou extends DontInheritMe {
Say(){
console.log("상속을 플렉스 했지뭐야~");
super.Say();
}
}
const saltDda = new IWillIngeriteYou();
saltDda.Say();
- Public, Private, Protected 지정자
- 갸~아 꿀 개꿀
- 이거 자바스크립트로 구현하려 해봐! 얼마나 짜증나는지!
- 우리 착한 컴파일러는 잘못 된 걸 그냥 넘어가지 않아요!
// public & protected
class ComputerCase {
protected orner : string;
public on(){
console.log('start bootloader...');
}
}
// private
class Computer extends ComputerCase {
private memory : string = "32GB" // 내 맥북 32GB 였으면 좋겠다.
private os : string;
constructor(os : string){
super();
this.os = os;
this.orner = 'Wayne';
}
}
let MyComputer = new Computer("mac").on();
//new Computer("linux").os; // 착한 타입스크립트 컴파일러는 그냥 넘어가지 않아요!
- readonly
- 아 설명이 필요한가?!
- gettter/setter
- 드디어 손쉽게~ 개 같은 할당으로 일어나는 거지 같은 일을 예방 할 수 있다.
class DontAssignDireclty {
private _high : number = 175;
get high() : number {
return this._high;
}
set high(high : number){
if(high < 175)
this._high = high;
else
console.log('더 크는 건 더 이상 불가능해! 인정해라');
}
}
let me = new DontAssignDireclty();
me.high = 183;
console.log( ` ${me.high} 야 `);
- static (정적 프로퍼티)
- 이게 무슨 설명이 더 필요한가?
- 추상클래스
- 다른 클래스가 파생될 수 있는 기본 클래스
- 안 그래도 추상적인 클래스를 레일 추상적으로 선언해서 인스턴스도 못 하게 만들어버린다.
- 야야 내가 봤을 때는 이 클래스는 요따구로 생겨야해! 참고해서 함! 만들어봐!
abstract class GirlFriend {
private face : string = "존예";
appear(){
console.log(this.face);
}
}
class RealGirlFriend extends GirlFriend {
// 왜! 왜?! 왜?!!!
}
let MyGirlFriend = new RealGirlFriend();
MyGirlFriend.appear();
- 클래스를 인터페이스로 사용하기
- 자바스크립트의 클래스는 프로퍼티에 대해 적절한게 프로토타입으로 만드는 문법에 설탕을 뿌린 것 뿐이다.
- 야야~ 자바스크립트 한태 소금 뿌려~~
- 어차피 대충 읽고 속성이랑 메소드만 빼오면 되는 거니까 인스턴스로 확장해서 써
- 자바스크립트의 클래스는 프로퍼티에 대해 적절한게 프로토타입으로 만드는 문법에 설탕을 뿌린 것 뿐이다.
'Javascript > Typescript' 카테고리의 다른 글
타입스크립트 핸드북 - 데코레이터 (0) | 2020.02.01 |
---|---|
타입스크립트 튜토리얼 - 리액트 & 웹펙 묶기 (0) | 2020.01.30 |
타입스크립트 핸드북 - 인터페이스 (0) | 2020.01.29 |
타입스크립트 핸드북 - 변수선언 (0) | 2020.01.29 |
타입스크립트 핸드북 - 기본 자료형 (0) | 2020.01.26 |